Using a ESP32 as the brains, a real time clock, and a 2.8″ TFT touchscreen, I set out to see if I could make a hydroponic garden controller. The TFT will allow the user to configure three channels, labelled Light, Fan, and Pump. The Light and Fan channels are Schedule based, and the pump is Duration based.
- ESP32 DOIT DEVKIT V1
- DS3231 Real Time Clock Module
- 2.8″ TFT Touchscreen featuring ILI9341
- (4) LEDs with current limiting resistors
The ESP32 will give us the speed needed to drive the TFT touchscreen display with a decent update rate, as well as add possibility to add Wi-Fi and Bluetooth in the future. Espressif (https://www.espressif.com/) has a large library of documentation and examples to use with the ESP-IDF (https://docs.espressif.com/projects/esp-idf/en/latest/esp32/get-started/index.html). But the ESP32 is also Arduino compatible, so let’s do our prototyping there for now.
For the real time clock, I am using the DS3231 on a breakout board with the RTClib (https://adafruit.github.io/RTClib/html/class_r_t_c___d_s3231.html) library. Robust library and easy to use, it uses I2C serial communication to get and set the current date time, and set any alarms. Adafruit has a great post about their breakout board (https://learn.adafruit.com/adafruit-ds3231-precision-rtc-breakout), that really helps to get started.
Finally, the 2.8″ TFT with ILI9341 iC driven the the Adafruit ILI9341 Arduino Library (https://github.com/adafruit/Adafruit_ILI9341) with the XPT2046 touchscreen controller using the XPT2046 Touchscreen Arduino Library (https://github.com/PaulStoffregen/XPT2046_Touchscreen) by Paul Stoffregen.
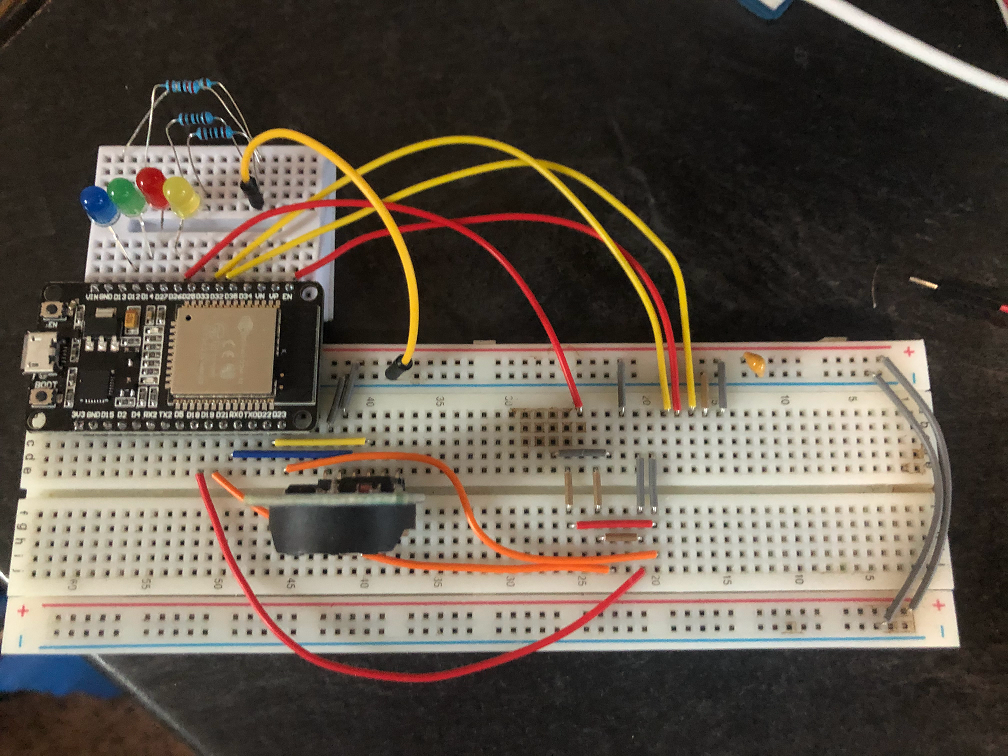
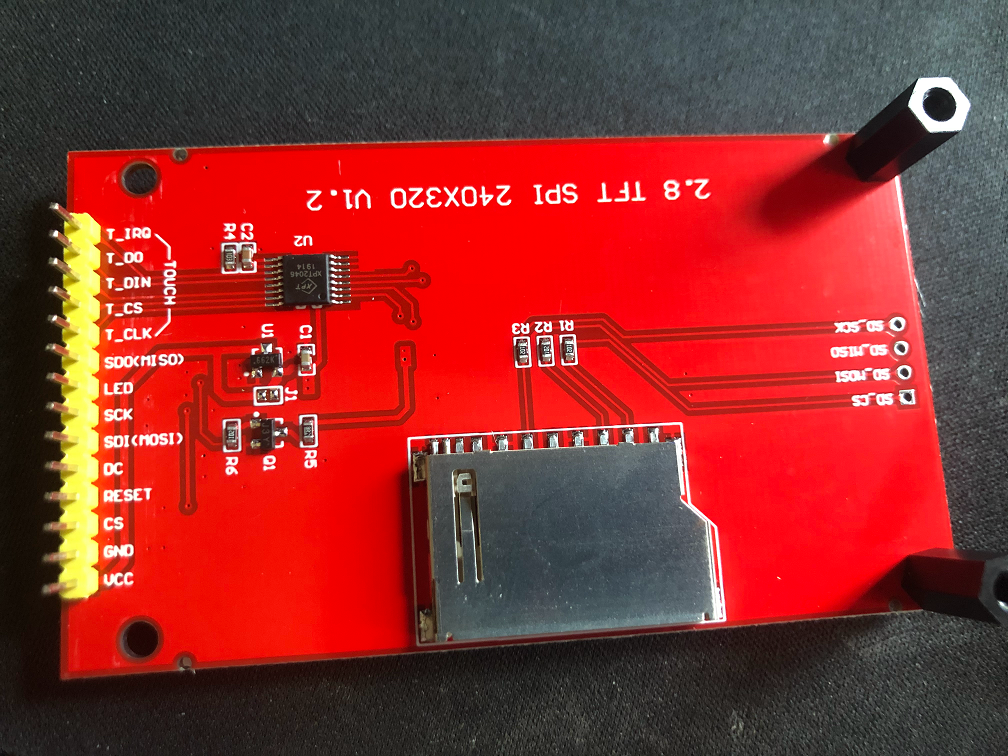
After connecting the EPS32 to the DS3231 via I2C pins, you can load up the RTClib example for the DS3231. This will allow you to verify everything is working correctly, and will set the correct time. The library uses the system date and time at the time of compilation and flashes that on to the iC. Here is an example of the rtc object being created before the call to setup, and the rtc calling the adjust method passing in the system date and time.
RTC_DS3231 rtc;
void setup() {
.
.
.
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
Now, any calls to rtc.now() return a DateTime object to use for any timekeeping activity. And when using the Alarm feature, you can trigger for multiple combinations of matching seconds, minutes, hours, and days.
For the touchscreen, there is a calibration sketch you’ll have to run for the screen rotation your using.
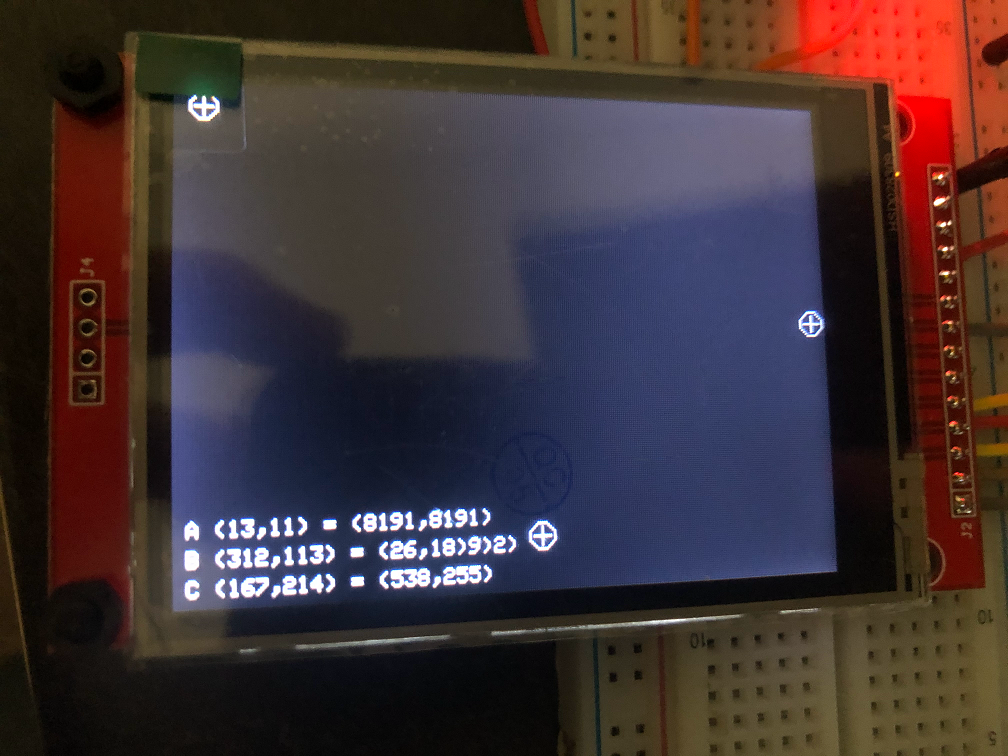
Now the screen is up and working, we can display basic information about the system in a text based format. Here is a video of the ESP32, real time clock, and 2.8″ TFT. The ESP32 polls the RTC’s alarm to see if the one second alarm has triggered. If it has, will run the block of code that requests the time from the RTC. Meanwhile, the TFT display will update portions of the screen, depending on where the “focus” is at that time.
Now it was about coding up a bunch of functions that each draw a different screen. We build a function to draw a particular screen depending on what is being done. Each screen will have corresponding buttons that only get “pushed” when we draw that screen.
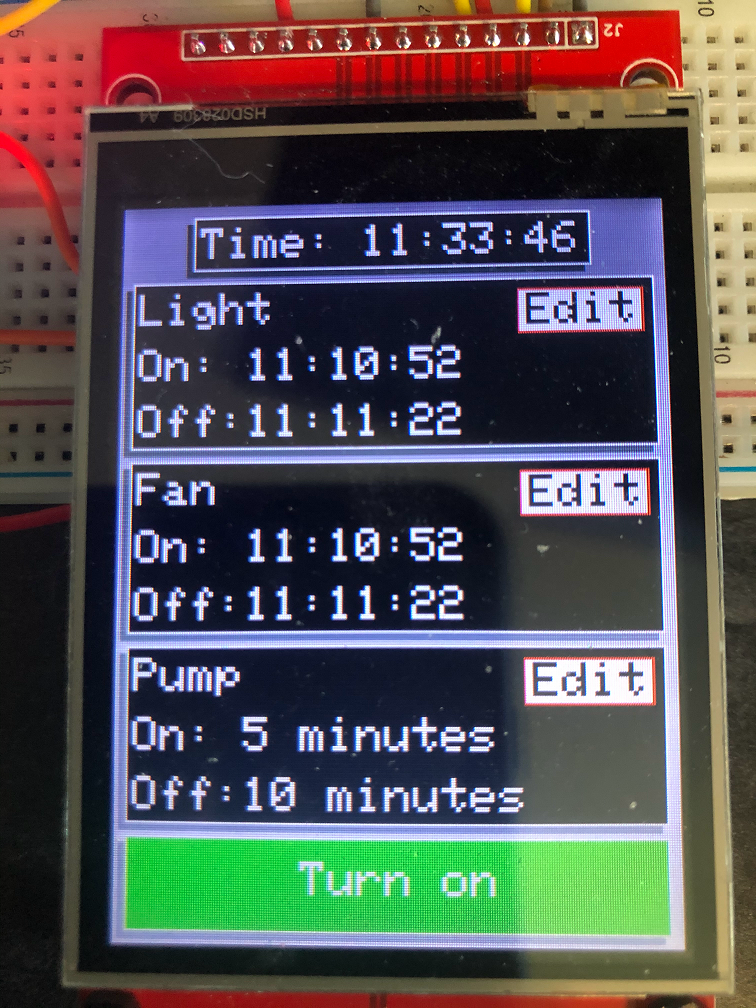
You can see the code I wrote this at my GibHub page: https://github.com/dpnebert/HydroController/blob/master/HydroController.ino
Things I would do differently for revision 2.0:
- Using the DS3231’s INT/SQW pin for triggering an interrupt on the ESP32 to avoid having to poll the alarm.
- Using the Strategy design pattern. Being able to add a new device to the list and change their implementation at run-time. Example would be adding a new Duration based device to channel 2. The Device objects can be stored as an array making it easy to iterate over all the objects.
Thank you so much for following along! I used a large piece of protoboard and some wires to create a more permeant solution, which can be found here: Protoboarded: Hydro Controller. Allowing me to move it around much easier while I continued to develop code for it, which that post can be found here: Coded: Hydro Controller Prototype. Please check out my recent posts to the right and thanks for following along!